The Form Token Field Gutenberg Component is used for creating an input field from which you get a list of suggestions (autocomplete) from which you select values. This is also used in the Tags field when you’re writing a post (or any similar taxonomy).
This component is a bit complex. If you’re going to read the source code, you might find yourself going deeper and deeper into other various components and classes. I’ll try to make it a bit easier for you to understand it with this tutorial.
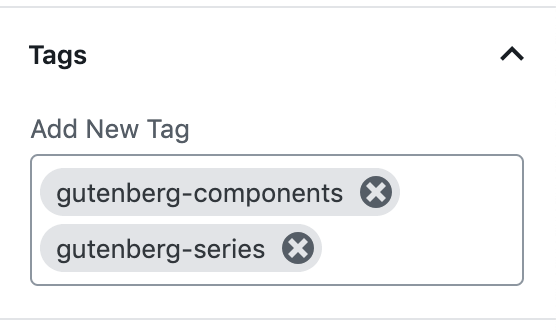
Before we look into how
value
– this is the property that accepts the tags that are going to be shown as selected. It can be an array of strings or an array of objects. If it’s an array of objects, the objects need to have the attributevalue
as well,displayTransform
– this property is a function that will transform the tags. By default, it uses the function identity fromlodash ,saveTransform
– this is also a function that is called when the tags are saved or when we need to match the suggestions with the current value. By default, it trims the tags,onChange
– this is a function that is called when we enter a new tag (when the values should be saved),onInputChange
– this is triggered when the type a tag in the input field. It accepts whatever we write in the input,onFocus
– this is triggered when the input is focused and the value passed is the whole event,suggestions
– an array of strings that will be used for suggestions that can be displayed based on the typed value,maxSuggestions
– a number for how many tags can be displayed to choose from,tokenizeOnSpace
– if true, when you press space (and there is something in the input), it will insert it,isBorderless
– if true, the tags will have aclass is-borderless
, maxLength
–number on how many tags can be chosen,disabled
– if true, tags can’t be removed or added,placeholder
– if passed, the input field will show a placeholder if there are no tags selected.
The objects in the property value
(if used), can contain:
value
– required,title
,status
– error, validating or success,onMouseEnter
– function,onMouseLeave
– function.
The Gutenberg Component From Token Field uses 3 other components (classes) to build the functionality:
Token
– each selected tag,TokenInput
– input where we type tags to add (or search),SuggestionsList
– list that shows the tags.
All of those components can be found in files from the Form Token Field repository folder.
How Gutenberg uses Form Token Field
Let’s now see how this component is utilized in Gutenberg to create the Tags field. The Tags field does not use the Form Token Field directly, but it actually implements it into another component. That component is FlatTermSelector from the
Let’s go over each property that FlatTermSelector is using with Form Token Field:
value
– selected terms are provided here,suggestions
– array of term names that can be chosen,onChange
,onInputChange
,maxSuggestions
– 20,disabled
– it is disabled while loading terms,label
– Label used for the text to add a new term,messages
– object with messages foradded
,removed
andremove
.
How the Terms are retrieved
The value
is populated from the variable selectedTerms
. These terms are retrieved from the state. If there is a property terms
passed to the FlatTermSelector, it will use those. Otherwise, it will fetch them from the taxonomy rest route for the post (or custom post type) that we are using.
When the terms are fetched, they are stored in the state of the component as availableTerms
. This is done like that because we want all the information on each term so we can manipulate them.
Then, the selectedTerms
availableTerms
The onInputChange
is also a function that will fetch the terms based on the value in the input. The function fetchTerms
will then again populate the suggestion list.
Building the Suggestions
The property suggestions
is populated from the variable termNames
. That variable is built from the availableTerms
.
How Tags are Saved
To save the tags, the FlatTermSelector component uses the property onChange
. The function used here receives the selected term names.
Since the database relationship between the post and taxonomy is through IDs, that function is building an array of term IDs based on the (previously stored) availableTerms
property from the state.
Then it passes all those IDs on the function that will update the terms (through the REST API).
Using the FormTokenField to retrieve Posts from a WordPress site
Let’s now use the Gutenberg Component FormTokenField to load posts and choose some of them. We won’t save them to any database, we will only use the local session storage of your browser. You can then refactor that method to do what you need it to do.
First, start a new React App using the “Create React App” by typing this in your terminal (command prompt). Be sure to position yourself in a folder you want to build this.
npx create-react-app my-app
Now place yourself in that folder and then let’s install the dependencies we need:
npm install lodash @wordpress/components @wordpress/api-fetch --save
We will need lodash
for a part where we save our posts that we retrieve. The other WordPress dependencies are what we need to use this component. The ApiFetch dependency is going to be used to retrieve the posts from your site.
Creating our Custom Component
Place yourself inside of the src
folder and create a components
folder and then a JavaScript file and give it a name you want. I’ve called it FormTokenField.js
because we are learning that.
Let’s add our dependencies first in that file.
Our component will be called SearchPosts and it will initial have a state with tokens
and availablePosts
. Both are arrays where the first one will hold post titles of selected posts and the second one will hold the data of all available posts that were retrieved from the site.
Searching for Posts
We will need a method that will be called when the input has changed and that will search for posts.
We are also binding this method in the constructor so we can use this
inside of it. For this tutorial, I’ve used my own site to retrieve posts but you can put any other site that has the REST API enabled.
This method will be passed on the onInputChange
property.
Saving & Retrieving Posts
We will save posts when a new post is selected. This method will be passed on onChange
The selected posts (tokens) are going to be saved in the state of the component and also in the local session.
When the component is mounted, we are checking the local session for stored post titles and then we are setting that as an array of tokens. In a real application, you would also fetch all the posts with such titles and store it in the state of this application (in availablePosts
for example).
Rendering the FormTokenField Component
Now we need to finish our component with a render method and export it by default so we can use it in other places of our application.
For the suggestions, we are building an array of post titles from the availablePosts
array. Then we are using the FormTokenField component and set the properties as we need them.
Rendering it in the App
We can now render our component in the App. Open App.js
src
Using the Styles from Gutenberg
This part is available only to the members. If you want to become a member and support my work go to this link and subscribe: Become a Member
Small Performance/UX Tip
This is just a small tip on how to load the posts and keep the component from loading more until the initial load is finished. We will utilize the disabled
property for that.
This part is available only to the members. If you want to become a member and support my work go to this link and subscribe: Become a Member
Conclusion
The Gutenberg component Form Token Field is a great component if you’re looking for something more than just an autocomplete solution. This field can be used when you want your users to pick up some categories, interests and various data that can be seen as a tagging data.
Become a Sponsor
Share this: