WooCommerce Subscriptions is a complex but excellent extension for WooCommerce stores if they plan to sell subscriptions. Sometimes, you want to have simple products but also sell the same as a subscription. In this tutorial series, we will allow the store to sell Simple Products as WooCommerce Subscriptions.
Some of the examples where you could use such solution:
- Selling a course with one-time price ($100) and an installment plan ($20/month for 6 months),
- Selling one magazine or a magazine subscription.
In this first part on this series, we will create the base of the plugin and the admin side of it. We will have to hook into the WooCommerce Product tabs and add subscription data.
Plugin Base
Our plugin for selling simple products as WooCommerce Subscriptions will be namespaced. You can name it however you want and the starter folder and file can be as well your own choice.
I’ll create a new folder under wp-content/plugins
and name it ibenic-simple-product-subscription
. I’ll also create a file inside of it with the same name ibenic-simple-product-subscription.php
.
Create another folder inside of it and name it includes
. Add this code there:
<?php
/**
* Plugin Name: Simple Product Subscriptions
* Description: Enable Simple Products to be sold as subscriptions.
* Version: 1.0.0.
* Author: Igor Benić
* Author URI: https://www.ibenic.com
*/
namespace Simple_Product_Subscriptions;
if ( ! defined( 'ABSPATH' ) ) {
return;
}
include_once 'includes/class-plugin.php';
$plugin = new Plugin();
$plugin->init();
Add now another file inside of includes
folder and name it class-plugin.php
. Put this code in it:
<?php
/**
* Main Plugin file. This class is used to instantiate and start the whole plugin.
*/
namespace Simple_Product_Subscriptions;
/**
* Class Plugin
*
* @package Simple_Product_Subscriptions
*/
class Plugin {
/**
* Initialize the Plugin
*/
public function init() {
add_action( 'plugins_loaded', array( $this, 'run' ) );
}
/**
* Run the plugin
*/
public function run() {
if ( ! class_exists('WC_Subscriptions' ) ) {
return;
}
include_once 'class-product.php';
if ( is_admin() ) {
include_once 'class-admin.php';
$admin = new Admin();
$admin->run();
}
}
}
In this part we are running the plugin inside of the hook plugins_loaded
. This means that all the main plugin files were loaded. That way, we are 100% sure that WC_Subscriptions
will exist if the plugin WooCommerce Subscriptions is active.
Product Class for Subscription Data
Before we start selling Simple Products as WooCommerce Subscriptions, we need to store:
- Subscription Period (day, week, month, year),
- Subscription Interval and
- Subscription Price.
Create a new file class-product.php
inside of the includes
folder and add the code below.
<?php
namespace Simple_Product_Subscriptions;
class Product {
/**
* Get Billing Subscription Interval
*
* @param integer $product
*/
public static function get_subscription_interval( $product ) {
return get_post_meta( $product, '_subscription_interval', true );
}
/**
* Get Billing Subscription Period
*
* @param integer $product
*/
public static function get_subscription_period( $product ) {
return get_post_meta( $product, '_subscription_period', true );
}
/**
* Get Subscription Price
*
* @param integer $product
*/
public static function get_subscription_price( $product ) {
return get_post_meta( $product, '_subscription_price', true );
}
/**
* Set Billing Subscription Interval
*
* @param integer $product
* @param mixed $value
*/
public static function set_subscription_interval( $product, $value ) {
return update_post_meta( $product, '_subscription_interval', $value );
}
/**
* Get Billing Subscription Period
*
* @param integer $product
* @param mixed $value
*/
public static function set_subscription_period( $product, $value ) {
return update_post_meta( $product, '_subscription_period', $value );
}
/**
* Get Subscription Price
*
* @param integer $product
* @param mixed $value
*/
public static function set_subscription_price( $product, $value ) {
return update_post_meta( $product, '_subscription_price', $value );
}
}
We can then use this class to easily retrieve subscription data for a particular product or to save such data. We will use that both on the admin and front side.
I have decided to go with the update_post_meta
and get_post_meta
functions. If we are to get the WC_Product object, we could use the methods get_meta
or update_meta_data
.
Displaying Simple Product Subscription fields
Let’s now display the fields on the admin side on Simple Products.
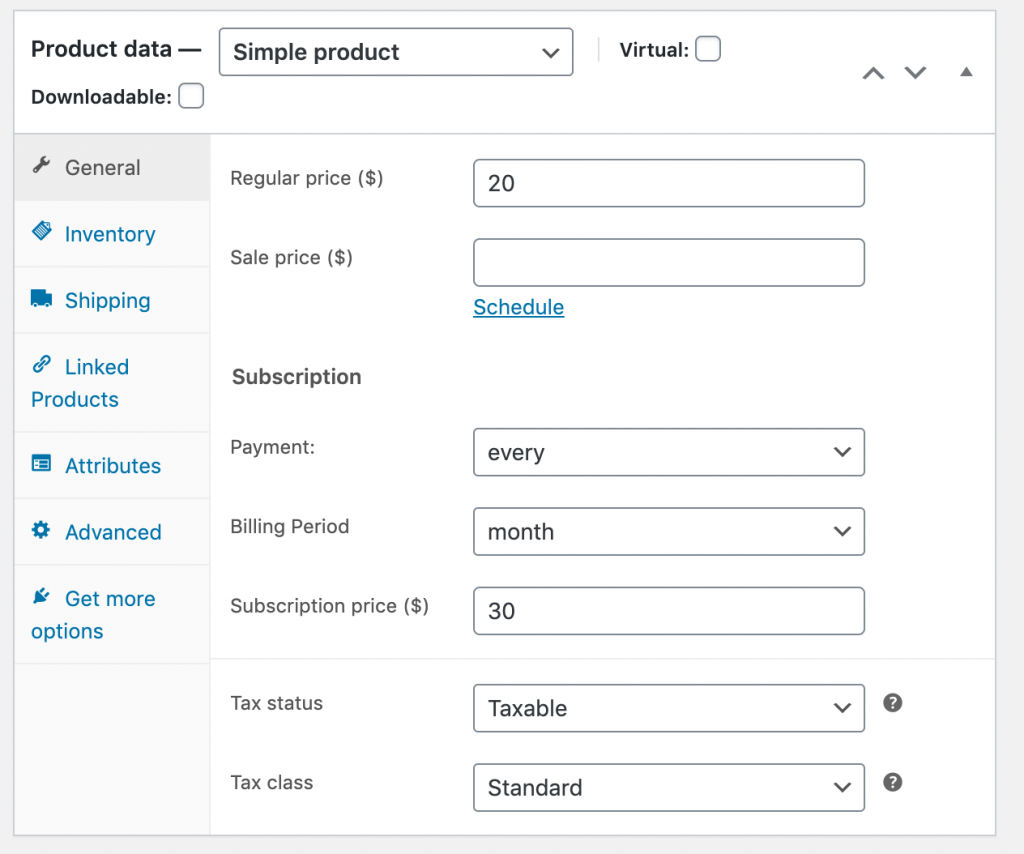
Let’s create a new file class-admin.php
inside of the includes
folder.
Displaying Fields
Add this code in that file to display the fields for subscriptions.
<?php
namespace Simple_Product_Subscriptions;
class Admin {
public function run() {
add_action( 'woocommerce_product_options_pricing', array( $this, 'display_subscription_options' ) );
}
/**
* Display Subscription Options
*/
public function display_subscription_options() {
global $product_object;
if( ! $product_object ) {
return;
}
if ( 'simple' !== $product_object->get_type() ) {
return;
}
echo '<h4 style="padding: 0 1em;">' .esc_html__( 'Subscription', 'simple-product-subscriptions' ) . '</h4>';
echo woocommerce_wp_select( array(
'id' => '_sp_subscription_interval',
'label' => __( 'Payment:', 'woocommerce-subscriptions' ),
'value' => Product::get_subscription_interval( $product_object->get_id() ),
'options' => wcs_get_subscription_period_interval_strings(),
)
);
// Billing Period
echo woocommerce_wp_select( array(
'id' => '_sp_subscription_period',
'label' => __( 'Billing Period', 'woocommerce-subscriptions' ),
'value' => Product::get_subscription_period( $product_object->get_id() ),
'options' => wcs_get_subscription_period_strings(),
)
);
echo woocommerce_wp_text_input(
array(
'id' => '_sp_subscription_price',
'value' => Product::get_subscription_price( $product_object->get_id() ),
'label' => __( 'Subscription price', 'woocommerce' ) . ' (' . get_woocommerce_currency_symbol() . ')',
'data_type' => 'price',
)
);
}
}
This code will now display the three fields for selling Simple Products as WooCommerce Subscriptions. You can now choose the interval of payments and the period along with the price that will be charged.
Saving Subscription Data
Let’s now also save the subscription data that we enter in those fields. We will write the code inside of the same class-admin.php
file.
In this code we are checking if our data is set inside of the $_POST
. If they are set, the data is saved to that product.
Code
The current code of this plugin can be download here so you don’t have to do everything on your own.
This part is available only to the members. If you want to become a member and support my work go to this link and subscribe: Become a Member
Conclusion
By the end of this tutorial, we were able to show fields for simple products only and save them if they exist when posted.
With that data we are mirroring the data that WooCommerce Subscriptions use when selling subscriptions. In the next tutorial on selling simple products as WooCommerce Subscriptions we will show the subscription button.
This button will be used to add it to the cart and use it as subscription.
Have you ever tried selling subscriptions with WooCommerce subscriptions? What were your challenges?
Share this: